Wednesday, December 28, 2005
Paging in SQL
Consider you want to find the records from 21-30 in order of their IDs, then you can do this using the folllowing query:
SELECT TOP pagesize *FROM (SELECT TOP (pagesize*pagenumber) * FROM tableName ORDER BY ID)
ORDER BY ID DESC.
Yup it is a very easy and handy way to implement paging.
Monday, December 19, 2005
Validating numeric data type
bool Validate(string text)
{
Regex reg=new Regex(@"\D");
return !reg.Match(text).Success;
}
Here if text contains only digits then the resull of reg.Match is false, so Validate will return true and vice versa. Here decimal point is also considered as non digit and will cause the result of the function to be false.
Now the following code snippet will recognize the period "." as the part of the digits also but more than one periods will not cause error i.e 123.45.67 will also return true.
bool Validate(string text)
{
Regex reg=new Regex(@"[^0-9.]");
return !reg.Match(text).Success;
}
Sunday, November 27, 2005
Windows Live
Windows Live consists of eight so Web-based services designed for customers. It consists of services like mail, online-messenger, online folder sharing, Internet telephony etc.
The most striking examples of Windows Live is ways of of tying it with desktop. People could share files-folders with the instant-messaging buddies and use the ww.Live.com page to view not only the Web-contents but also the things like recently opened documents or corporate SharePoint portal.
Live.com and Gadgets
Although customizing Live.com is not as easy as it should be due to skills and steps required to add cutom gadgets to it but the personalization features offered btu it are far more than that of Yahoo or even Google. Imagine you can use same point to view your corporate portal from home, access files from your office PC in another country or even check mails, send messages to your buddies and share file-folders with them. All from same point. Microsoft has promised to ensure that adding gadgets will be as easy as dragging and dropping a link on a site but at the moment it is not as easy. People have to go to microsoftgargets.com, copy a special URL, then go back to Live.com and follow a series of "advanced options". Gadgets has an important place in Windows Vista and Windows Live as Microsoft plans to use them thorughout them with no difference. The same gadget can also be used in Windows Vista. Microsoft also plans to use gadgets to retrieve locally stored information from PC to Live.com Web-page. Gadgets are actually small applications like Web-parts but they mainly consist of XML-based manifest file, JavaScript (containing entry point) and CSS file. Live.com uses Atlas framework to extend JavaScript functionality. Live.com is designed to take things one step further. It allows people not only to view Web contents and personalize them but also to save search queries as well as data from their PCs.
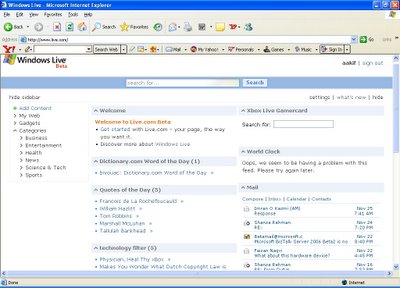
Windows Live Mail
Windows Live mail is an extension of hotmail codenames KAHUNA. It provides a new style of interface, a more desktop like mail software, with features including automatic inbox refreshing, spell-checking, phishsing detection and a preview pane that will enable hotmail users to read and respond to thier e-mails without ever leaving their inboxes. Its interface in known internally as "WAVE 11" and it is designed to be lightweight and clean for better performance. It provides preview to your e-mail without loading a new page each time (like Outlook®) drag and drop messages into folders, and generally power through your e-mail in a flash.
Windows Live Spaces
Windows Live Spaces is a transition of MSN spaces with some added functionality.
Windows Live Messenger
Some of the biggest new thing that Microsoft has introduced in Windows Live is Windows Live Messenger. The new messenger has additional features like social networking, Internet telephony, folder sharing etc. Folder sharing is really a fantastic idea. You just have to drop a file on top of a contact which will allow you to create a shared folder. This folder will exist on both members' desktop and stay up-to-date with any changes to the shared file.
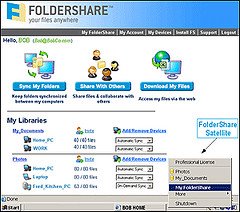
Windows OneCore Live
It is a security system that helps you protect your system. It includes features like virus scanning, firewall settings, software backups and tune ups.
Windows Live Search
It includes elements of Microsoft's Virtual Earth mapping. It allows members or their buddies to creat annotations, creating a personalized map of their favorite spots in the city, even from your mobile, plus you can see detailed maps, driving directions and more.
Saturday, November 26, 2005
Technical Interview Questions - Part 2
Yes, it is possible if you want to prevent its instantiation or if you want to have a singleton class.
Q.2 What is the purpose of singleton pattern?
To manage accesses to a shared resource.
Q.3 Is it possible that we can inherit singleton class?
A singleton class cannot be inherited i.e. a class having its ocnstructors private including the default constructor cannot be inherited. It will generate a compile time error.
Q.4 Can we implement inheritence (polymorphism) using delegates?
Yes we can implement polymorphism using delegates i.e. inheritance. A delegate can be attached to any function having same signature.
Q.5 Can we use delegates in place of inheritence (polymorphism)?
Nops, we cannot do this as delegates can be attached to any function having same signature but in case of inheritance only methods of the same class or its parent class can be called.
Q.6 Which is faster, stored procedures or embedded SQL?
Stored procedures as they are pre-compiled, optimized and stored with the database. On the other hand embeded SQL is compiled every-time when it is executed.
Lazy Instantiation and the Singleton Pattern
Lazy Instantiation lets the creation of objects be put off until they are actually needed i.e. they are created on need. Then what is its relation with singleton pattern. This is actuall what it is required in singleton pattern. Here I want to point out one more thing that lazy instantiation is closely related with the static members. The .Net compiler deals with the static members in the same way. They are created when any member (static or instance) member of the class is first referenced in the Application Domain and remain alived until the AppDomain exists but I think the big plus of using static members is that they are thread-safe and optimized by the constructor of .Net.
Usually we implement singleton-pattern using the following code:
public sealed class Singleton
{
static Singleton instance=null;
Singleton()
{ }
public static Singleton Instance
{
get
{
if (instance==null)
{
instance = new Singleton();
}
return instance;
}
}
}
but the above code is not thread-safe as two threads can simultaneously check the status of instance variable and both can enter into critical section. Another way of doing this is to use locking like :
public sealed class Singleton
{
static Singleton instance=null;
Singleton()
{ }
public static Singleton Instance
{
get
{
lock (instance)
{
if (instance==null)
{
instance = new Singleton();
}
return instance;
}
}
}
}
but it will cause performace loss as at one time only one thread can be in critical section and having instance check outside the critical section will cause the same problem. A simple solution of the above problem is to declare and define the static instance of the object at the same time like:
public sealed class Singleton
{
static readonly Singleton instance=new Singleton();
// Explicit static constructor to tell C# compiler not to mark type as beforefieldinit
static Singleton()
{ }
Singleton()
{ }
public static Singleton Instance
{
get
{
return instance;
}
}
}
Now here a question arises. Why I have used static constructor when nothing is done in it? Well the secret of using it is the laziness of type initializers is only guaranteed by .NET when the type isn't marked with a special flag called beforefieldinit. Unfortunately, the C# compiler (as provided in the .NET 1.1 runtime, at least) marks all types which don't have a static constructor (i.e. a block which looks like a constructor but is marked static) as beforefieldinit. So to use static members in place of lazy instantiation, the class must have static constructor, otherwise ins laziness is not guaranteed.
Another thing, if the class has another static member, in that case also the laziness of the class is not guaranteed.
Tuesday, November 22, 2005
Technical Interview Questions
Well I have seen many people get confused in this question and telling differences as:
1 -- An abstract class is a class which cannot be instantiated and an interface is a set of functions. Thier usage would be goverened by the follwoing reasons :-a. If I have some common functions to be implemeted in the base class then one should use abstract class as Interface cannot have funcation implementations.b. Languages like C# and VB.NET only allow single inheritance of classes hence you have only one base class and rest will have to be created as interfaces.e.g. If I did my class A to inherit from Class B and Class C .. then inheritance can only be done from one of these classes the one will have to created as an interface.
c. Interfaces cannot have state information i.e. they cannot contain variables but can contain constants. On the other hand, an abstract class can contain both variables and constants.
All these answers are right but one has missed the key answer. A child class implements interface but extends abstract class.
What it actually means is that an interface is a contract between 2 or more classes i.e. it defines mutual agreement between 2 or more parties like interfaces exposed for COM objects.
On the other hand an abstract class defines a relationship with its child class not the contractual relationship but inheritance. It is a IS-A relationship between abstract class and child class.
So if you want to implement generalization/specialization i.e. HAS-A relationship then use abstract class but if you want to provide a common interface or contract between 2 or more parties so that service-consumer class only needs to know the services of its service-provider class not their implementation use Interfaces.
Q.2 Can you have two applications on the same machine one which is using .NET Framework 1.0 and the other using 1.1 ?
Yes
Q.3 If you are using a web service and you want its path to be picked up from the config file what will you do ?
Set its behaviour property to dynamic this will add a new appSetting entry in the config file for the webservice path.
Q.4 Can I serialize Hash table with XmlSerializer?
XmlSerializer will refuse to serialize instances of any class that implements IDictionary, e.g. Hash table. Soap Formatter and Binary Formatter do not have this restriction.
Q.5 Whether ADO.Net provides conneted recordset like ADO or dis-connected?
ADO.Net provides both types of recordsets. A connected recordset is called data-reader but using data-reader we cannot modify database using it. It is useful for small number of data-records as it is very fast but useless for large number of records as it will keep the connection with tha data-base for a long number of time. We can extract records from multiple tables i.e. multiple record-sets in the same data-reader.
A dis-connected record-set is called a DataSet. It is a complete mirror of data-base i.e. we even can keep relationships in it. A data-set can contain multiple tables with relationships among them. User can write the contents of the dataset in an XML file without any effort. A data-set can also be used to move data from one database to another.
Q.6 What does 'managed' mean in the .NET context?
The term 'managed' is the cause of much confusion. It is used in various places within .NET, meaning slightly different things.
Managed code: The .NET framework provides several core run-time services to the programs that run within it - for example exception handling and security. For these services to work, the code must provide a minimum level of information to the runtime. Such code is called managed code. All C# and Visual Basic.NET code is managed by default. VS7 C++ code is not managed by default, but the compiler can produce managed code by specifying a command-line switch (/com+).
Managed data: This is data that is allocated and de-allocated by the .NET runtime's garbage collector. C# and VB.NET data is always managed. VS7 C++ data is unmanaged by default, even when using the /com+ switch, but it can be marked as managed using the __gc keyword.
Managed classes: This is usually referred to in the context of Managed Extensions (ME) for C++. When using ME C++, a class can be marked with the __gc keyword. As the name suggests, this means that the memory for instances of the class is managed by the garbage collector, but it also means more than that. The class becomes a fully paid-up member of the .NET community with the benefits and restrictions that brings. An example of a benefit is proper interop with classes written in other languages - for example, a managed C++ class can inherit from a VB class. An example of a restriction is that a managed class can only inherit from one base class.
Q.7 What is reflection?
All .NET compilers produce metadata about the types defined in the modules they produce. This metadata is packaged along with the module (modules in turn are packaged together in assemblies), and can be accessed by a mechanism called reflection. The System.Reflection namespace contains classes that can be used to interrogate the types for a module/assembly.
Using reflection to access .NET metadata is very similar to using ITypeLib/ITypeInfo to access type library data in COM, and it is used for similar purposes - e.g. determining data type, their sizes for marshaling data across context/process/machine boundaries.Reflection can also be used to dynamically invoke methods (see System.Type.InvokeMember), or even create types dynamically at run-time (see System.Reflection.Emit.TypeBuilder).
Reflection can also be used for late-binding,r producing generic methods or mosly for dynamically generating classes and modules. Remarkable, isnt it.
Q.8 What is the difference between Method Hiding and Shadowing?
Well there is no difference in these concepts.They are just 2 sides of the same coin, 2 names of the same term but in different technologies. Method hiding is the concept of C# whereas method shadowing is the concept of VB.Net. Method Hiding and Shadowing are the concepts by which you can provide a new implementation for the base class member without overriding the member. It is similar to method overriding but only in this case you are hiding and shadowing the definition of the parent class. For both these concepts same rules apply as in case of Method overrding i.e. the signature of the method must be same.
Now what is the difference between Method hiding and Shadowing with Method overloading. Hmm, it is simple. The difference lies when you call the derived class object with a base class variable.In class of overriding although you assign a derived class object to base class variable it will call the derived class function. In case of shadowing or hiding the base class function will be called.
To shadow a member of the parent in child class, use Shadow keyword in VB.Net and to hide a member of the parent class in child, use new keyword in C#.
The following is the code snippent, showing these concepts:
Method Shadowing:
Public Class BaseShadow
Public Overridable Function OverrideMe() As String
Return "Base Class Override Me"
End Function
Public Function ShadowMe() As String
Return "Base Class Shadow Me"
End Function
End Class
Public Class DerivedShadow Inherits BaseShadow
Public Overrides Function OverrideMe() As String
Return "Derived Class Override Me"
End Function
Public Shadows Function ShadowMe() As String
Return "Derived Class Shadow Me"
End Function
End Class
Method Hiding:
public class BaseHide
{
public BaseHide()
{ }
public virtual string OverrideMe()
{
return "Base Class Override Me";
}
public string HideMe()
{
return "Base Class Hide Me";
}
}
public class DerivedHide:BaseHide
{
public DerivedHide()
{ }
public override string OverrideMe()
{
return "Derived Class Override Me";
}
public new string HideMe()
{
return "Derived Class Hide Me";
}
}
Thursday, November 17, 2005
MSN decides to jump into Net-calling future
The move comes as all the major portal and IM companies are moving more heavily into Internet calling. This move comes after the launch of Googles IM Google Talk, which focus on voice chatting. But Microsoft further added that it will be paid service even in its beta state.
For details: http://news.com.com/MSN+buys+into+Net-calling+future/2100-1032_3-5844873.html?tag=nl
Some Useful Network Commands
The following are some useful DOS commands that helps you monitor your netwok:
1. net user -- List users on your machine
2. net user /domain -- Lists all the users on the domain. If no domain is specified after /domain switch then it will get all the users from the first directory of the forest.
3. net user /domain username -- Lists the entire active directory entry for the user username from the active directory.
4. net view -- Lists all the active machines on the domain.
5. net view \\machineName -- Lists all the shares on the machine specified. It is optional whether to use its dns name or IP address.
6. net use \\machineName\IPC$ "" /u:"" -- Connects to the Inter Process Communication Share IPC$ on the remote machine (machineName) with the built-in anonymous user. The connection can only be established if the NetBIOS protocol is enabled on the remote machine and port 139 is not blocked.
7. net stat -- List all the open ports on your machine and their status.
8. nbtstat -A ipAddress -- Dumps the NetBIOS table of the remote machine.
9. arp -A -- Lists all the entries of your MAC table and their types.
10. route -- With the help of this command you can modify the routing table i.e. add, modify or delete all the gateway entries. e.g. route ADD 157.0.0.0 MASK 255.0.0.0 157.55.80.1 METRIC 3 IF 2 destination^ ^mask ^gateway metric^ ^Interface. If IF is not given, it tries to find the best interface for a given gateway.
11. route print -- Lists the routing table.
Inter-Process Communication
Well have you ever think of interprocess communication between two different types of processes e.g. one process of Java and second process of .Net. Well there are many ways to accomplish this using different technologies and tools namely:
1. XML Web Services
2. Remoting
3. MSMQ
4. COM communication (DCOM)
5. e-Connects like BizTalkServer etc.
but what if we dont want to rely on any extenal technology or tool like MSMQ, COM or e-Connects then I think XML Web Services is the best choice for you. You can use Remoting as well but it requires some server for communication. XML Web services is the techology that lets you communicate between processes without having to write a large piece of code or using any external technology. Just expose the service provider process as XML Web Service and you can consume its services from the consumer. Hence no external component is required for inter-process communication.
Sunday, November 13, 2005
Email using CDOSYS
It is really easy to create a mail using the COM object of CDO.SYS. An instance of the COM object can be created in Sql in the similar fashion as in C++ using the sp_OACreate stored procedure. This procedure takes 2 parameters, one is a string containing the name of the object to be created and second is the reference to the object to be created and returned. After calling this stored procedure, its second parameter contains the reference to that COM object. This procedure returns the status that can be used for error handling.
The second step is to setup the properties for this COM object which can be setup using the schema from microsoft server such as smtp server to be used and the store procedure sp_OASetProperty. Sendusing field specifies whether to use the port 25 or some other method. The value 2 represents port 25. Other fields can also be set here such as smtpconnectiontimeout etc. After setting up all these fields one should also call the update method of the cdosys COM object which can be called using the sp_OAMethod store procedure.
After setting up all these fields, one should set the headers of the mail also namely To, From, Date, and Subject. Other headers can also be set similarly.
Finally set the Body of the mail. It can be either Text or HTML. Separate properties are available for setting up either body type but only one can be used at one time. Finally call the Send method of the COM object to send the mail. This method can be called in the similar fashion as Update method for configuring fields.
It is better to clear the COM object after use. This can be done using the store procedure
sp_OADestroy and passing the COM object to be destroyed.
I think C++ developers will find it quite similir to what they usually do in C++. I have provide the SQL version of the CDOSYS emailer.
SQL Version
CREATE PROCEDURE [dbo].[xp_cdosendmail]
@From varchar(100) ,
@To varchar(100) ,
@Subject varchar(100)=" ",
@Body varchar(4000) ="
AS
Declare @Msg int
Declare @status int
Declare @source varchar(255)
Declare @description varchar(500)
Declare @output varchar(1000)
--************* Create the CDO.Message Object ************************
EXEC @status = sp_OACreate 'CDO.Message', @Msg OUT
--***************Configuring the Message Object ******************
-- This is to configure a remote SMTP server.
EXEC @status = sp_OASetProperty @Msg, 'Configuration.fields"http://schemas.microsoft.com/cdo/configuration/
sendusing").Value','2'
-- This is to configure the Server Name or IP address.
-- Replace MailServerName by the name or IP of your SMTP Server.
EXEC @status = sp_OASetProperty @Msg, 'Configuration.fields("http://www.blogger.com/, 'luckymail'
-- Save the configurations to the message object.
EXEC @status = sp_OAMethod @Msg, 'Configuration.Fields.Update', null
-- Set the e-mail parameters.
EXEC @status = sp_OASetProperty @Msg, 'To', @To
EXEC @status = sp_OASetProperty @Msg, 'From', @From
EXEC @status = sp_OASetProperty @Msg, 'Subject', @Subject
-- If you are using HTML e-mail, use 'HTMLBody' instead of 'TextBody'.
EXEC @status = sp_OASetProperty @Msg, 'TextBody', @Body
EXEC @status = sp_OAMethod @Msg, 'Send', NULL
-- Sample error handling.
IF @status <>0
select @status
BEGIN
EXEC @status = sp_OAGetErrorInfo NULL, @source OUT, @description OUT
IF @status = 0
BEGIN
SELECT @output = ' Source: ' + @source
RaisError( @output , 16, 1)
SELECT @output = ' Description: ' + @description
RaisError( @output , 16, 1)
END
ELSE
BEGIN
RaisError(' sp_OAGetErrorInfo failed.', 16, 1)
Return
END
END
-- Do some error handling after each step if you have to.
-- Clean up the objects created.
EXEC @status = sp_OADestroy @Msg
What is the purpose of our lives
Here another question arises, if we need to acquire our natural resources then we cant do it alone. We need interaction with others i.e. there should a social code of conduct. There comes religion-a true social and economical code of conduct. All the dealing between 2 or more people require some governace to ensure a fair dealing. Here comes some socio-cultural responsibilities. But the person who is governing this also needs some assistance for his/her livings and he is not the only person who is providing social services. All these men/women require also their livings. Also to provide these people livings there must be some economical system to gather money from people using these sercvices. Hence a cycle is established and all we get trapped into this cycle. Then what happens to our purpose. Our pose is to manage all these socio, economical and cultural reponsisbilities fairly and honestly and use our choices for good not for grant and waste them.
We have been given intelligence so that we can manage and organize all these social, economical and religious responsibilities efficiently without getting indulged in any wrong and always made right and fair choice, dont go for rationale choices as they may lead you on wrong steps.
But a real men/women would do all these responsibilities so fairly and efficiently that he/she will be remembered after on and I think in which ever field we are engaged we should try to be high and be prominent in it. How by hard, honest and efficient working so that we can be remembered after on.
I think it is enough for this time:)
Wednesday, November 09, 2005
How to handle New Row in the Grid Control
So I used the following code for the detached row and it worked:
//If it is a new row that has just been added but not attached to data grid, then ends its editing.
if(dr.RowState == DataRowState.Detached )
this.BindingContext[this.grid.DataSource, this.grid.DataMember].EndCurrentEdit();
A generic Equal Method
public override bool Equals(object obj)
{
PropertyInfo [] properties=this.GetType().GetProperties(BindingFlags.Instance BindingFlags.Public BindingFlags.NonPublic);
foreach( PropertyInfo property in properties )
{
object obj1=property.GetValue(this, null), obj2=property.GetValue(obj, null);
if( (obj1==null && obj2!=null) (obj1!=null && obj2==null)
((obj1!=null && obj2!=null) && !obj1.Equals(obj2)))
return false;
}
return true;
}
public override int GetHashCode()
{
return base.GetHashCode ();
}
Copy constructor for child object
A a=new B();
Where A is the child object and B is the parent which is not possible. We can achieve this goal by copy constructor like this:
public BaseEntity(ref object parent):this()
{
if(parent==null)
return;
FieldInfo [] fields;
if(parent.GetType()==this.GetType().BaseType)
fields=parent.GetType().GetFields(BindingFlags.Instance BindingFlags.Public BindingFlags.NonPublic);
else
fields=this.GetType().GetFields(BindingFlags.Instance BindingFlags.Public BindingFlags.NonPublic);
foreach(FieldInfo field in fields)
{
object val=field.GetValue(parent);
field.SetValue(this, val);
}
}
It works like:
B b=new B();
A a=new A(b);
How to find the path of the layouts folder of SPS using IIS interface
private string FindSPSPath( string spsPath )
{
try
{
string metabasePath="IIS://Localhost/W3SVC";
DirectoryEntry path = new DirectoryEntry(metabasePath);
PropertyCollection properties=path.Properties;
DirectoryEntries entries=path.Children;
foreach(DirectoryEntry entry in entries)
{
if( entry.SchemaClassName=="IIsWebServer" && entry.Properties["ServerComment"][0].ToString().ToLower().Equals(spsPath.ToLower()) )
{
DirectoryEntries vdirs = entry.Children;
foreach(DirectoryEntry vdir in vdirs)
if( vdir.Name== "root")
{
DirectoryEntries childVdirs = vdir.Children;
foreach(DirectoryEntry childVdir in childVdirs)
if(childVdir.Name=="_layouts")
return childVdir.Properties["Path"].Value.ToString();
}
}
}
return null;
}
catch
{
throw;
}
}
Here what I have done is to traverse through the collection of children of the IIS Server i.e. IIsWebService. It contains all the Web Servers and Application Pools indicated by a number n following the path IIS://Localhost/W3SVC/n. The next step is to find the appropriate Web Server you are looking for which can be found through the ServerComment property of the Web Server. Also dont forget to check the type of the child of IIS as it can be application pool and application pools dont have such property. The SchemaClassName of the Web Servers is IIsWebServer.
Now we have found the Web Server of the SPS (SharePoint Portal Server). The next step is to find the layouts virtual directory of SPS. Here is something that is typical to SPS. The SPS conatins 2 virtual directories which are not visible through inetmgr that are root and filters. root is the virtual directory that we are interested in as it contains all the rest virtual directories including _vti_bin, layouts etc. So we have now reached to layouts virtual directory of SPS. The final step is to find its physical path. The Path property of virtual directories contain their physical path. In the similar fashion you can find any property of any virtual directory or can modify them.
Remoting Configuration
RemotingConfiguration.Configure("validate.config");
but if you dont want to hard code the name of the configuration file and want to use the default config file of your application. How you can achieve this. Well it caused me a lot of time to find it but finally it is really easy. Simply use the following code:
RemotingConfiguration.Configure(AppDomain.CurrentDomain.SetupInformation.ConfigurationFile);
How to create a VB Script Object and call methods on it
Dim IIsWebServiceObj Set IIsWebServiceObj = GetObject("IIS://localhost/W3SVC")
IIsWebServiceObj.DeleteExtensionFileRecord "E:\RMS\Code\BusinessServices\KalSPS\Debug\KalSPS.dll"
To write this code in C#, you just need to add a reference to Microsoft.VisualBasic.CompilerServices and System.Runtime.CompilerServices. The following code snippet creates an IIS object and call DeleteExtensionRecord Method on it.
private void RemovesWebServiceExtension(string extensionPath)
{
try
{
object ext = this.GetIISObject("IIS://" + Environment.MachineName+"/W3SVC");
object[] args=new object[1]{extensionPath};
LateBinding.LateCall(ext, null, "DeleteExtensionFileRecord", args, null, null);
}
catch
{
throw;
}
}
private object GetIISObject(string fullObjectPath)
{
try
{
return RuntimeHelpers.GetObjectValue(Interaction.GetObject(fullObjectPath, null));
}
catch
{
throw;
}
}
where Interaction module contains procedures to interact with objects, applications and system. Runtime Helper class contains method for compiler construction. It is a service class and hence contains only static methods. Its GetObjectValue method boxes the object passed as the parameter. Boxing a value type creates an object and performs a shallow copy of the fields of the specified value type into the new object. Finally LateBinding is used as an object of type Object can be bound to object of any type i.e. it behaves as a varient type.
The first argument of LateBinding.LateCall method is the object on which the method should be called, second argument is the type of the object i.e. System.Type object, the third argument is the name of the method, 4th is set of arguments to be passed to that method, 5th argument is the set of names of the parameters to be passed to that method if they have any, and finally 6th argument is the set of booleans indicating whether to copy back any parameters.
Tell me isnt it very simple